Bash is a Unix and Linux shell and command interface language. The bash was released in 1989 with the GNU project. The Bash became popular with Linux and related distributions like Ubuntu, Debian, CentOS, RHEL, etc. Bash provides a very useful command-line interface with complete scripting language features. Linux commands can be executed via the bash command-line interface. Also, the bash scripting features can be used to create simple to complex scripts and programs. In this tutorial, we examine how to create and run a bash script.
Check Your Shell Type
Every Unix and Linux shell provides the $SHELL
environment variable. This $SHELL environment variable stores the current shell executable file which also displayed the current shell. This $SHELL environment variables can be displayed with the following echo
command.
$ echo $SHELL
/bin/bash
From the output, we can see that the current shell executable is /bin/bash
which means this shell is Bash. If the shell is different than the Bash the bash shell script may or may not work which is unreliable.
Create Bash Script File
Creating a bash script file is easy as creating a regular or text file. There are different ways and tools to create a script file. First, we use the nano
text editor in order to create a bash script file. Nano is a user-friendly text editor which runs on the bash command-line interface. We provide the bash script file name to the nano text editor as a parameter. In this example, we set the file name as backup.sh
.
$ nano backup.sh
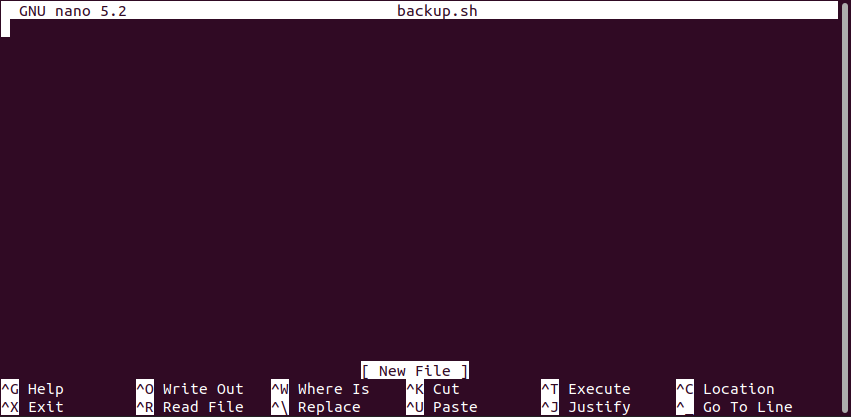
As a general rule, every script file should start with the interpreter binary location. As this is a bash script file the interpreter is a bash interpreter or executable which is located /bin/bash
. Also, we add the #!
signs at the start of the interpreter to depict it.
#!/bin/bash
Say “Hello World”
We have created the bash script file and added the interpreter to the start of the bash script. Now we make a conventional start which is “Hello World” which is the most popular application in the world for all programming and scripting languages. We print the “Hello World” to the standard output which is the command-line interface.
#!/bin/bash
echo "Hello World"
Press CTRL+C in order to save and exit from the nano text editor. After pressing CTRL+C it asks to “Save modified buffer”. Type “Y” and press enter to save and exit.
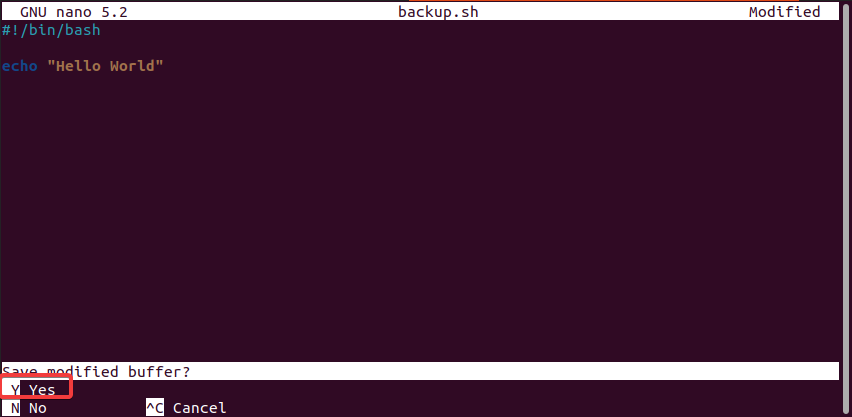
Execute The Bash Script
Now we have created the bash script file and put some script inside it. The script file is named as baskup.sh
. We can execute this script file. The most basic way to run a bash script file is using the bash
command or binary and provide the script file name as a parameter.
$ bash backup.sh
Alternatively, we can specify the bash script file absolute of full path to call it from the different working directories.
$ bash /home/ismail/backup.sh
The best way to run a bash script is to make it executable with the chmod
command like below. After making the bash script file executable we can directly call it to execute. There is no need to use the bash command.
$ chmod u+x backup.sh
$ ./backup.sh
Create and Use Variables
Bash provides variables in order to store different types of values like string, character, number, etc. The variable can be defined, created, and set by typing the variable name and assigning it the value we want to set. The equal sign is used for the assignment. The bash variable assignment is like below.
VARIABLE=VALUE
- VARIABLE is the variable name we want to set.
- VALUE is the value of the variable.
Variables can be used with the $
prefix. For example below we create the variable named age
and use it $age
.
#!/bin/bash
name="ismail"
age=37
echo "My name is $name"
echo "I am $age years old"
When we executed this bash script the output is like below.
My name is ismail I am 37 years old
Run Commands In Bash Script
Like the bash command-line interface, we can run commands in a bash script and use their outputs. In order to run a command, the command put inside the $(COMMAND)
where the COMMAND is the command we want to run.
#!/bin/bash
cwd=$(pwd)
current_user=$(whoami)
echo "Current working directory is $cwd"
echo "Current user is $current_user"
Current working directory is /home/ismail/nmap Current user is ismail
Take User Input
The bash script can be used to take user input interactively during the execution of the bash script. The read
command is used to read user input and set it into the specified variable. The syntax of the read command is like below.
read VARIABLE
In the following example we ask the user’s name and age, then print it to the screen.
#!/bin/bash
echo "What is your name?"
read name
echo "What is your age?"
read age
echo "Your name is $name."
echo "You are $age years old."
